- Skip to primary navigation
- Skip to main content
- Skip to primary sidebar
- Skip to footer


How To Use the TripAdvisor API to Build a Simple Travel App
By Team RapidAPI // September 14, 2020
Let’s create an “Explore the World!” web app with TripAdvisor API. I’ll be showing you how to create the web app from ideation to implementation, and walking you through each step of the way with diagrams, explanations, and code samples.
Let’s get started!
Connect to the Tripadvisor API
How to Build a Simple Travel App
The idea behind the simple web app is to call and get corresponding information from the TripAdvisor API regarding three important trip-planning categories: flights , hotels , and attractions , and then saving the trip itinerary in our database to be retrieved at a later time.
Sounds complicated? It’s okay! Let’s take it one step at a time.
Here is a sketch of the web app that I’m envisioning. This can be done on Figma (free), Sketch, or Adobe XD but, in a jiffy, I just grabbed some pens and after a few revisions, sketched this:

I’m going to redo the sketch in Figma in the next tutorial, but in the meantime, I took a look at the documentation for the TripAdvisor API and am going to request an API key . An API key is a string of characters that allows you to uniquely call and access the information provided by the API, provided to you, the developer, by the TripAdvisor API. This allows the API to keep track of who is making the calls, what and how many calls are being made. There are a few hoops to jump through for this, so I’m going to focus on requesting and getting the API key before going back to working on the design any further.
According to the TripAdvisor API documentation, getting a key might be a wait, and impossible without having a website setup ( https://developer-tripadvisor.com/content-api/request-api-access/ ). So now, my todo’s are to quickly set up a static website and have a good description of the intended use of the TripAdvisor API to justify why I should be getting an API key.
We’ll be creating something similar to this:

This is a static page that has some letters on it. We will deploy this page using my favorite web app deployment tool: Heroku .
Side plug for Heroku, a piece of third-party software: It’s a great website that allows the user to quickly deploy a web app with a unique domain and fix bugs on the web app according to the Heroku logs. It’s command-line friendly and is a quick way to deploy your web app to the world wide web.
Moving forward, the technology stack we’ll be using for the static website and for the working version of the later website is a Node development environment, with Node, Express, MongoDB, and Handlebars. I’ll go into each of those briefly in the following table:
For this tutorial, we’ll just focus on doing the bare-bones of a web app, meaning we’ll just set up Node, Express, and Handlebars (and wait for the next tutorial to explain saving the itinerary into a database).
The first iteration of the functionality of the web app will be to make one type of API call to the TripAdvisor API and display that in on a page that is readable for the user.
Here are the three views that you will create in this particular tutorial, plus one more which we’ll go over later:

Step 2. Set up Node, Express, and Handlebars
To make the three static pages above, we’re going to have to install Node.js , Express.js , and Handlebars.js. In the table above, there is a link to instructions and information for the set of each, but in a nutshell, you will need to open your terminal, create a folder and use the commands “npm init”, “npm install express”, and “npm install express-handlebars”
If you are already familiar with using Node , Express, and Handlebars, skip onto Part III: Add Some Basic Routes and Handlebars. If not, stick with me for a little longer!
Okay, so once we have installed the three packages, we will make a file called “server.js” using the command “touch server.js” in the folder that you made. This may be the most important file you make for a Node development environment.
Server.js is the entry file that when node runs, it goes to. A sample “Hello World” server.js file looks like this:

There are a few important parts of the above code. The term ‘require’ imports the express engine into our file. When we save that as a constant, we can use the express engine wherever we need to. In addition, our first route is defined by ‘/‘, which will send the words ‘Hello World’ to the defined port ‘3000’. App.listen is a method that sends information to the defined port, which happens to be ‘3000’.
At this point, if you “npm start server.js” in the terminal, and then go to https:// localhost://3000 in your browser, you should see the words: Hello World.
Step 3. Add Some Basic Routes and Handlebars
Getting your first route to work and show up on the browser is quite exciting, I have fond memories of mine first “Hello World” page. But! Let’s move onto something a bit more exciting: rendering templates for text, images, and colors.
Here is the directory hierarchy I used for my templates:

Starting from the top, there is an image of the camera that I used in the header, with a reference for where the image came from the page. Underneath, and here is where the directory hierarchy is most important, is “layouts”, “partials”, which includes the “footer” and “navbar”, and the “about”, “attractions”, “contact”, and “home” page. It is of utmost importance that the hierarchy is written in this way because that is how Handlebars templating works.
I won’t go into the details (CSS) about each of the pages, instead, I’ll link them here for you to peruse at your own leisure.
Link: https://github.com/thecodingsophist/explore-the-world/tree/master/views
Step 4. Get the API Key
In the meantime, I’ve heard back from TripAdvisor, and they decided to not give me an API key, which, fairly, according to their documentation, is only given out to websites that had a certain volume of site hits and had a certain level of legitimacy.

No matter, we can forge ahead with making calls using the RapidAPI TripAdvisor API key.
Go to the TripAdvisor API and test endpoints . The right side of the panels should return a code snippet that contains:
We’ll be using this snippet of code later. While you are on the TripAdvisor API documentation page, check out the different sections of code that are generated on the right panel by your options on the left, especially the ‘GET’ routes under ‘Shared’, ‘Restaurants’, ‘Attractions’, ‘Hotels’, and ‘Flights’.

Here, you begin to see the possibilities of what information can be returned and used in your web app.
Step 5. Plan a Route
As you can see, there are some parameters that required and some are optional when you make an API call . For our web app, we will make a page that renders a list of 5 top attractions when you enter a city into a form on the “home” page of the web app.
Navigate to the “GET attractions/list” endpoint requirements on RapidAPI. Here, we see that the required parameters are just one, which is the location_id. This is an id for each location that TripAdvisor has an entry for.

We can just follow instructions underneath the red box, which is that “The value of location_id field that returned in locations/search endpoint”. This directs us to make a call under GET locations/search in order to return a location_id, which we can then use to add to the required parameters to return the attractions associated with that location or location_id.
Step 6. Code the Route
Now that we’ve seen a high level of how to obtain the information we need to make a call, let’s actually make the call!
In views, we’ve created a homepage that has a form which “GET”s to the route “/location”. The input type is “text”, and the id is “location”.

We can read this in our /location controller, under the controllers “directory”. If you open the file here , at the top, you see the line:

The variable location is being read using the method “param” associated with “req”. In short, “req.param” reads the URL being set by the location id from the form in the first code snippet in this section. We can now change the query to RapidAPI to the name of the location that was entered in the form. Check this query out:

The variable location, which was set to the location read in from the form on the homepage, will be used to find the location_id.
In short, we are using the location variable to query the string for the location_id, which we will then use to get a list of attractions from for that location_id.
Next, we use the variable “options”, defined earlier, in our request call from the TripAdvisor API:

In the sample code above, we save the returned object as “data”, which is parsed using the function JSON.parse(body). We then use locationId variable in our query for attractions, saving it as a field in variable options_for_loc.
Using the same request and response method, we can then use “attractions_data = JSON.parse(body)”, for when the request for attractions based on the locations_id comes back.
See code here .
Next, is just some data cleaning. We save the top 5 attractions into a list called attractions. We can prepare the data to pass through back into the attractions.handlebars template under the views directory.
To pass the information back into the attractions.handlebars template, we use the function: res.render(), where you pass through the destination, the main layout of the template, and your saved variables.

From the ‘attractions.handlebars’ point of view, all we have to do is access the variables passed through using {{ }}.
An example being:

And voila! That is how you pass information from a form, send a request based on the form’s entry, and then send another request based on the result of the first request using TripAdvisor’s API.
Step 7. Celebrate! And GET back to coding…
In the future, I will write about how to book hotels and flights through the TripAdvisor API, and use more advanced CSS, like the original vision, but for now, you are welcome to play around and check out the live website that this tutorial is written for:
https://explore-the-world-with-me.herokuapp.com/
Team RapidAPI
Reader interactions, leave a reply.
Your email address will not be published. Required fields are marked *
- API Courses
- API Glossary
- API Testing
- API Management
- Most Popular APIs
- Free APIs List
- How to use an API
- Learn REST API
- Build API’s
- Write for Us
- API Directory
- Privacy Policy
- Terms of Use
Building an Enterprise API Program Learn More
Go to list of users who liked

Extracting Information from TripAdvisor: A Guide to Scraping Data from Hotels and Restaurants
- #TripAdvisorDataScraping
- #ScrapeTripAdvisorData
- #TripAdvisorReviewsScraper
- #TripAdvisorReviewsScraping
- #TripAdvisorDataCollection
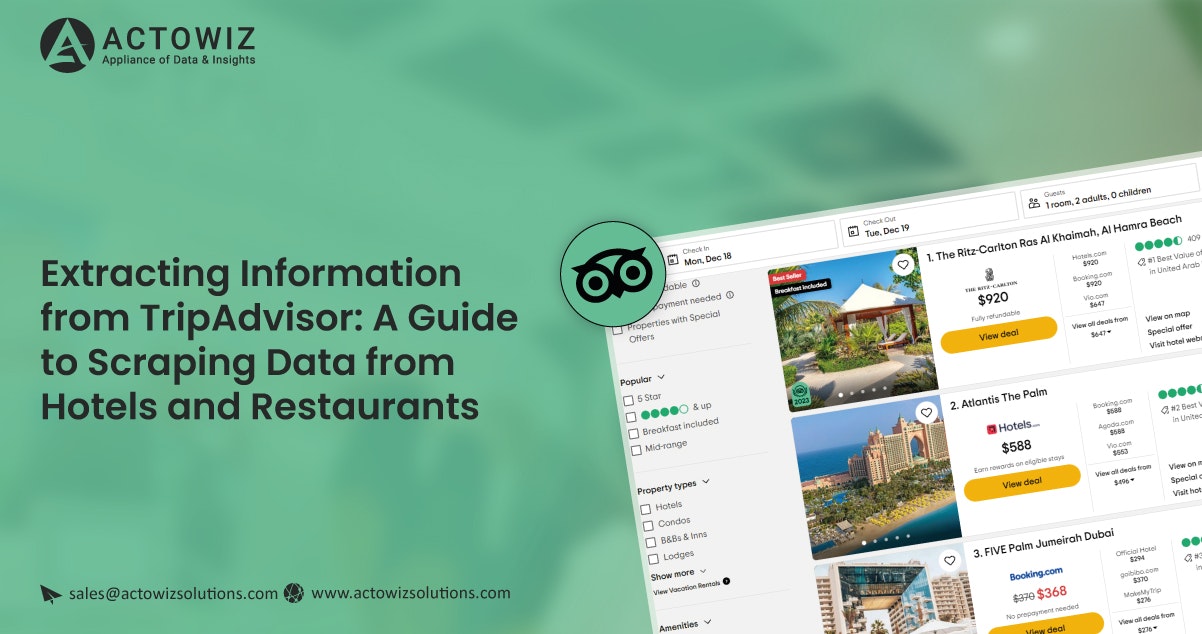
Extracting-Information-from-TripAdvisor-A-Guide-to-Scraping-Data-from-Hotels-and-Restaurants Introduction Leveraging the appropriate API makes scraping TripAdvisor on a large scale a straightforward task. You don't need to possess advanced computer skills to achieve this. Our comprehensive, step-by-step guide is designed to walk you through extracting data from TripAdvisor using a user-friendly web scraping tool.
TripAdvisor stands out as a powerhouse with an extensive database comprising over 8 million locations, 1 billion reviews, and support for 29 languages. As of 2022, when the cumulative reviews surpassed the one billion mark, it became evident that TripAdvisor's meticulous gaze would soon cover every restaurant, hotel, vacation rental, or attraction listing.
Unlocking the Potential: The Advantages of Scraping TripAdvisor Data Amidst TripAdvisor's vast sea of information lies a treasure trove awaiting extraction, analysis, and innovative presentation. For those in the tourism, hospitality, or travel sectors, harnessing TripAdvisor data proves invaluable for monitoring competitors and supporting strategic business decisions. The TripAdvisor Scraper facilitates seamless and rapid web scraping, offering the most straightforward route to consistently obtaining data at scale. This article delves into the myriad benefits of scraping TripAdvisor, shedding light on how this process can be initiated, including exploring the initial steps involving API utilization. Dive into the realm of data-driven insights with TripAdvisor scraping.
Unlocking-the-Potential-The-Advantages-of-Scraping-TripAdvisor-Data Demystifying the TripAdvisor Content API: Your Gateway to Seamless Data Integration The TripAdvisor Content API, formerly the TripAdvisor API, offers an official avenue for scraping TripAdvisor data. A significant evolution from previous years, the TripAdvisor Content API streamlines the process, requiring less rigorous vetting for access. Interested enthusiasts can explore various TripAdvisor APIs on the official platform. Unlike in the past, obtaining a key has become less restrictive, opening doors for developers to effortlessly tap into TripAdvisor’s wealth of data and seamlessly integrate it into their websites and applications.
This API provides access to diverse information, including details on accommodations, restaurants, and attractions. Users can extract valuable data such as review links, ratings, awards, accommodation categories, attraction types, and restaurant cuisines. The TripAdvisor Content API simplifies the extraction process. It empowers developers to enhance their platforms with real-time, relevant data from one of the most comprehensive global travel and hospitality databases. Dive into the world of data-driven development with the TripAdvisor Content API.
Navigating the Constraints of the TripAdvisor API: Considerations and Alternatives While the TripAdvisor API presents a convenient means to access data, notable limitations warrant careful consideration. One key constraint involves the restricted scope of data accessible through the API. If you've been following closely, the bullet list earlier merely scratches the surface of TripAdvisor’s comprehensive dataset. Crucial elements like vacation rentals, detailed restaurant reviews, activities, pricing information, itineraries, and addresses may not be readily available via the API, necessitating alternative approaches for comprehensive data extraction.
Moreover, the TripAdvisor API imposes specific restrictions on data volumes, introducing further complexities. These include limits such as extracting only up to 5 reviews and 5 photos per location, a monthly cap of 5,000 free API calls, a maximum of 10,000 calls per day even with payment, the allocation of only one API key per account, mandatory setting of a daily budget, and immediate provision of billing information. Additionally, meticulous monitoring of API usage is essential to prevent accidental overages.
In scenarios demanding extensive data beyond the API's thresholds, exploring alternative data sources or employing supplementary methods may be necessary to fulfill specific data requirements.
Navigating-the-Constraints-of-the-Tripadvisor Finally, while the API offers TripAdvisor data in an organized format, seamlessly incorporating this data into your website or application may pose challenges. It necessitates programming proficiency to manage API requests, parse the data, and present it in a user-friendly manner. This task can be more intricate than web scraping, where you have direct influence over data extraction and presentation. Now, let's explore how a straightforward scraper can be employed.
Why Should You Use TripAdvisor Scraper? The Tripadvisor Scraper offers a streamlined solution for large-scale data extraction, allowing users to download information in various structured formats like JSON, CSV, XML, or Excel files. Remarkably, no programming or coding skills are required to operate this tool. As an unofficial Tripadvisor API, it automates the extraction process, simplifying and expediting the scraping of Tripadvisor data. This efficiency allows users to focus on leveraging the extracted data to enhance and benefit their business without needing extensive technical expertise.
List of Data Fields List-of-Data-Fields Business Name Address Phone Number Website Email (if available) Category/Type of Business Overall Rating Number of Reviews Individual Review Ratings Reviewer's Username Review Date Latitude Longitude City Country Region Price Range Operating Hours Amenities Photos Popular Dishes/Services Wheelchair Accessibility Parking Availability Wi-Fi Availability Reservation Options Booking Website Links Discounts Promotions Links to Social Media Profiles Changes in Ratings Over Time Trends in Reviews Legal Compliance in Extracting TripAdvisor Data: Navigating the Terrain Extracting data from TripAdvisor is legally permissible due to its public nature. Scraping details from hotel pages aligns with accepted practices, but strict compliance with regulations like GDPR or CCPA is crucial, mainly when dealing with personal data like reviewer names. Caution must be exercised to avoid scraping copyrighted or private content, ensuring a responsible and lawful data extraction process.
How to Extract data from TripAdvisor? To initiate the process of data scraping from TripAdvisor, follow our simple 5-step guide using the TripAdvisor Scraper:
Step 1: Navigate to the TripAdvisor Scraper Page Click on the TripAdvisor Scraper page.
Press the ‘Get Started’ button.
Navigate-to-the-Tripadvisor-Scraper-Page Step 2: Select the Target Location for Scraping Select-the-Target-Location-for-Scraping Please provide start URLs to crawl.
You can change this later by going to your crawler>Settings>Start URLs Whether it's hotels, vacation rentals, restaurants, or attractions, you have the flexibility to gather information from any globa destination available on TripAdvisor
Just copy and paste the URLs from which you want to scrape data.
Just-copy-and-paste-the-URLs-from-which-you-want-to-scrape-data Press ‘Continue’.
Step 3: Initiate Scraping by Clicking Start Initiate-Scraping-by-Clicking-Start Simply click on the "Start" button and patiently await the results. The scraping process may take a few minutes.
Step 4: Retrieve Your Extracted Data Retrieve-Your-Extracted-Data Once your task is done, you will get the ‘Finished’ status and then you will be able to ‘View’ and ‘Download’ data in Excel (CSV), JSON, and XML format.
How to Extract TripAdvisor Reviews? If your goal is to specifically scrape reviews, consider using the TripAdvisor Reviews Scraper. This specialized tool allows you to gather valuable data for your analytics, including review title, text and URL, rating, published date, basic reviewer information, owner's response, place details, and more. Whether it's for restaurants, tourist attractions, hotels, or any other entity with reviews on TripAdvisor, this scraper is designed to capture relevant information.
You need to follow the same procedure discussed above for scraping TripAdvisor review data.
Contact Actowiz Solutions for more details. You can also reach us for all your mobile app scraping, instant data scraper and web scraping service requirements.
sources >> https://www.actowizsolutions.com/tripadvisor-scraping-guide-from-hotels-and-restaurants-data.php
Go to list of comments
Register as a new user and use Qiita more conveniently
- You get articles that match your needs
- You can efficiently read back useful information
- You can use dark theme
How to Scrape Reviews From TripAdvisor Using an Application Programming Interface (API)
TripAdvisor reviews contain a lot of helpful information about flight and hotel prices that can help you boost your business. It’s also home to tons of useful stats about popular travel destinations, hotels, and restaurants.
Table of Contents
- 1. Why TripAdvisor Reviews Are So Important
- 2. How Can Scraping Help You Collect Data From TripAdvisor Reviews?
- 3. Scraping Reviews From TripAdvisor With an API
- 4. How to Scrape TripAdvisor Reviews With an HTML Module
- 5. Other Ways to Scrape TripAdvisor Reviews
If you want to extract and use all of this data, you can use web scraping to automatically extract data from TripAdvisor reviews. Web scraping involves using automated bots to collect data from an HTML version of the site and deliver the collected data in Excel or CSV format, so you can process, analyze, and use the information.
As the most effective data collection method currently available, data scraping reviews from TripAdvisor will greatly boost your ability to synthesize, organize, and understand existing trends in the hospitality industry.
By using an application programming interface (API) or HTML module, you’ll be able to get the data you need smoothly and quickly. Read on to learn more about why TripAdvisor reviews are important, what scraping is, and how you can scrape reviews from TripAdvisor using an API or an HTML module. We’ll also walk you through how to use Scraping Robot’s API and HTML scraper.
Feel free to use the table of contents to skip to the section(s) that interest you the most.
Why TripAdvisor Reviews Are So Important
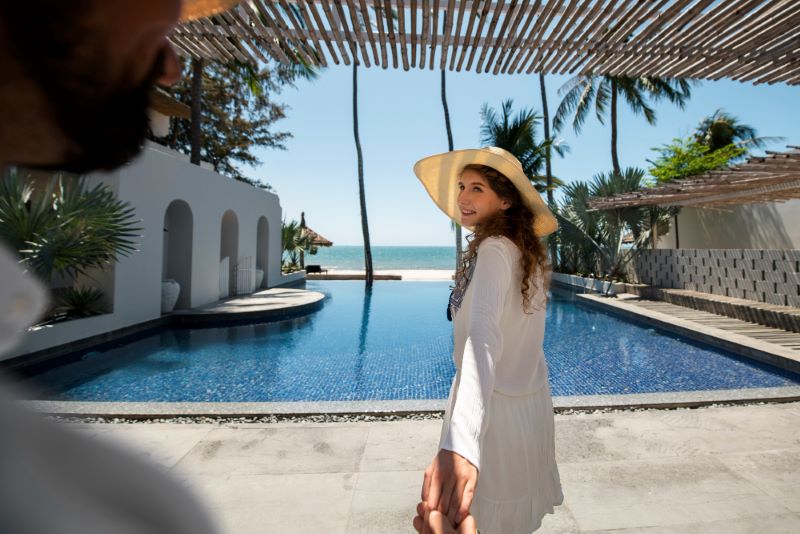
How many reviews are on TripAdvisor? As of 2020, TripAdvisor has over 884 million reviews of hotels, accommodations, and more.
This means that TripAdvisor reviews can tell us a lot about flights, facilities, experiences, and other things, which can help consumers:
- Get a better understanding of the most liked and most disliked tourist attractions in a particular area
- Obtain in-depth research about travel destinations
- Avoid common tourist mistakes or traps
- Discover new places, accommodations, and experiences
For instance, if you’re looking to get out of Seattle for a weekend, you can use scraping to discover which nearby city is the cheapest. Similarly, you can use scraping to spot and avoid common mistakes travelers often make when touring a particular area.
You should also consider looking through TripAdvisor reviews if you’re a travel and tourism company because it will help you:
- Understand your travel facility’s reputation and find room for improvement. Whether you’re running a tour of local wineries, a bed or breakfast, a motel, or a hotel, TripAdvisor review data will give you a sense of how the public sees your facility.
- Grasp the travel industry’s current trends and how you can catch up and stand out from your competitors.
How Can Scraping Help You Collect Data From TripAdvisor Reviews?
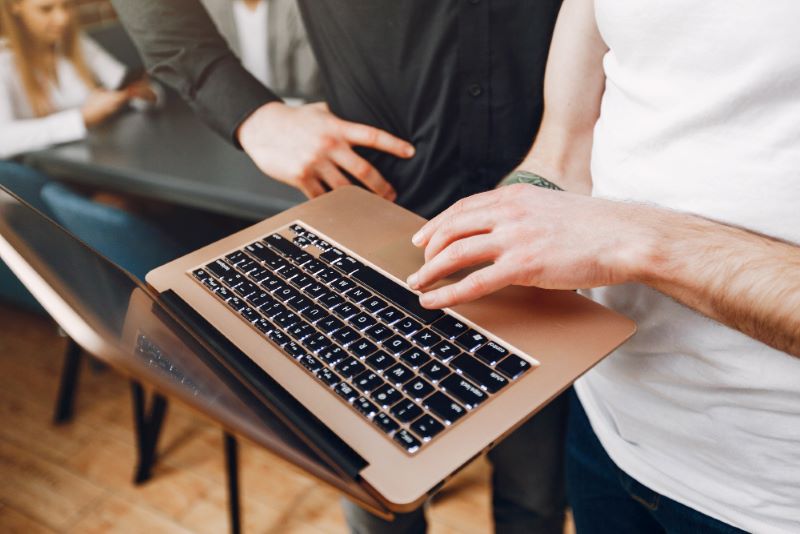
Scraping is the best way to access and use the massive amount of data contained in TripAdvisor reviews. A scraper will help you compile and transfer data into a spreadsheet for analysis and review.
Traditionally, you would have to go through each TripAdvisor review and manually input the different parameters into a spreadsheet. For example, when reading the TripAdvisor review below, you would note in your Excel sheet that:
- It’s a five-star review
- It was reviewed on February 5, 2020
- It was posted using a mobile phone
- The writer had visited this location in February 2020
- One person found this review helpful
From TripAdvisor
With scraping, you won’t have to take such detailed notes for every review. With just a couple of clicks, you’ll be able to determine which reviews were considered helpful by others and narrow your search results to specific areas or facilities you’re looking for. For example, you can find the best deals for different rental properties by scraping for nightly rates. You can also scrape to filter reviews by date and author.
Scraping Reviews From TripAdvisor With an API
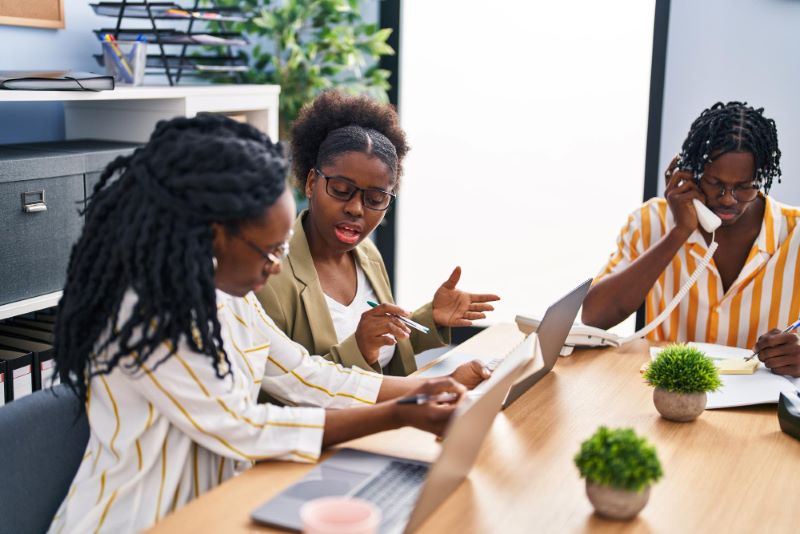
An API will allow you to scrape through web pages in real-time, instead of individually entering each page you want to scrape.
An API is a software interface that connects different computer programs and allows them to transfer data without exposing the code underlying each data transfer. By allowing data to be transferred from one software to another, an API will enable you to create a data funnel from your scraping software to the API to your data analytics software or database without any manual input.
APIs also allow you to extract and isolate categories of data so you don’t have to sift through a large amount of data in your database. This is how you can find your reviews on TripAdvisor and how you can get recent reviews on TripAdvisor. You can also use code to direct your API to send commands to your scraping software to extract specific categories of data from the pages you want, even when you aren’t at your computer. This will allow you to keep statistics on datasets that are always changing, such as stock market prices.
Additionally, APIs allow you to request data from web pages every 60 seconds, which you can then funnel into your own data projects, software, and other processes. Without an API, you would have to manually check up on these pages multiple times a day.
Although APIs seem complex, scraping TripAdvisor reviews with an API can be very simple.
Here’s how you can get started:
- Download and install your web scraping software. Make sure that you understand the software documentation before you install it. Note, however, that some APIs, like the Scraping Robot API , are browser-based and don’t need to be downloaded.
- Go to the web page you want to scrape.
- Copy the URL.
- Paste the URL into the program.
- You will then receive the full HTML output within seconds.
- Most web scraping tools have an extraction sequence for different HTML elements on a page. For instance, most scraping tools extract the text first. You can then select other extractable categories, such as Inner HTML, Class Attribute, JSON object, Captcha, href attribute, and full HTML. Available categories depend on what web scraping tool you’re using, so make sure that you got the right web scraping tool for what you want to achieve.
- Scraping Robot’s API simplifies this process by giving you all categories of HTML after you press the “run” button.
After receiving the full output, you can then export the data to your preferred analysis program, such as XLSTAT, Graphpad, and SPSS.
If you want to learn more about how Scraping Robot implemented the Scraping Robot API, check out our recent article .
How to Scrape TripAdvisor Reviews With an HTML Module
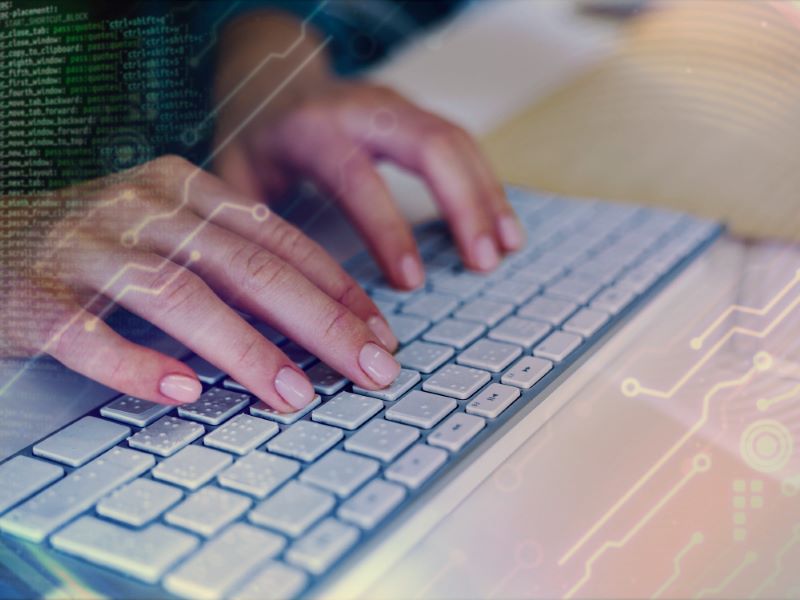
Although it’s highly recommended that you know how to code before using APIs, if you’re not a coder, you can still scrape TripAdvisor reviews using Scraping Robot’s pre-built HTML module.
To start scraping with this HTML module:
- Go to Scraping Robot’s HTML scraper, which will allow you to collect any kind of HTML data from any website.
- Copy the URL of the page you want to scrape and paste it into the URL field.
- Under the CSS selector field, paste in CSS selectors to look for elements to extract.
- Under the XPath field, paste in your XPath. XPath allows you to compute values or select notes from an HTML document. Play around with the XPath expressions to extract web data
- Press the “start scraping” button after checking off “I’m not a robot.” You should now get the HTML versions of the URLs you pasted in CSV format. Like Scraping Robot API, this HTML scraper allows you to collect any and all categories of HTML data.
- Download the CSV file or export it directly to your database.
If you want to scrape multiple pages, click the “add another row” button at the bottom to add up to 10 scrapes at a time. If you need more, you can sign up for a free trial.
Other Ways to Scrape TripAdvisor Reviews
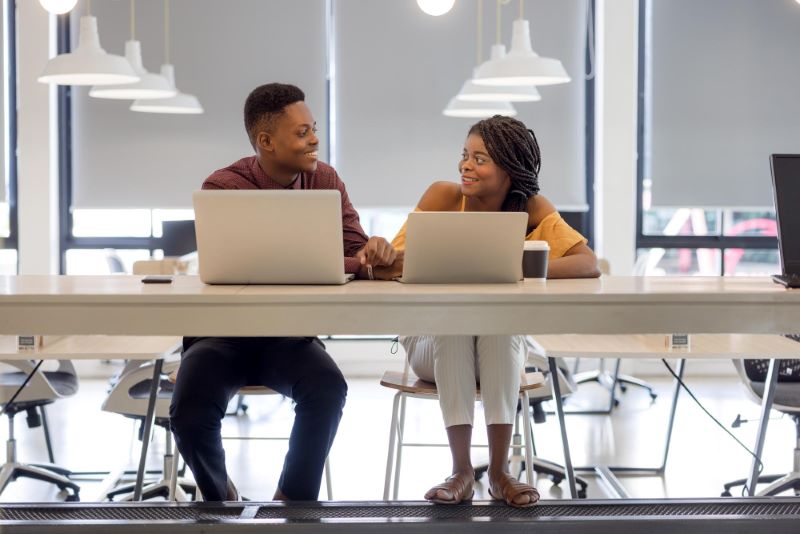
If the above solutions aren’t working for you, feel free to submit custom work to us at Scraping Robot by scrolling down to the “Contact” section.
You can also reach out to us if you have an idea for a new module. We are always looking for new ways to develop and improve our products, and we have great faith in the creativity and ingenuity of our customers.
TripAdvisor reviews are a great starting point for consumers and companies alike. As a consumer, scraping reviews will help you plan the ideal trip and avoid common mistakes. If you’re a travel agency or facility, data pulled from TripAdvisor reviews will make you aware of what clients are looking for, so you can stand shoulder to shoulder with your competitors.
Although learning how to scrape reviews from TripAdvisor sounds difficult, it’s actually quite easy if you use Scraping Robot’s browser-based API and HTML Scraper. Most scraping tools collect different categories of HTML data and need to be downloaded, but Scraping Robot’s API and HTML Scraper allow you to collect any and all categories of HTML data from all kinds of websites and set up a data funnel to populate your database. Both tools will save you a lot of stress and help you to arrange, manipulate, and organize data.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.
Related Articles
How to get Tripadvisor API access key and use it fast and easy
What is Tripadvisor API?
Tripadvisor API key is used to integrate the service content into a website or a mobile app, get reservations through the platform, and also direct your customers’ reviews to Trip. It provides several variants according to the applicant’s needs and requirements.
This kind of API provides Tripadvisor business ratings for free to be displayed on your website. But this is granted only to B2C websites after they’ve become approved partners. To become one, you have to fill in the form at Tripadvisor service and wait for a decision on your application.
This is a whole set of solutions for businesses working in the Hotel and Hospitality Industry. These API Tripadvisor keys help them to generate new bookings and collect reviews easily. This package also is aimed at so-called ‘Connectivity Partners’ – B2B internet services for the tourist industry.
This particular kind of API is used by the Tripadvisor approved partners (usually hotels, inns, and B&Bs) to show their prices and availability on the Tripadvisor website online. This API also allows users to book reservations right on Tripadvisor, without redirecting to a hotel landing page.

How to get Tripadvisor API key, credentials, and signature
If you want to get full access to Tripadvisor API solutions, you definitely need to become an approved partner and receive your API key. It might not be so easy and fast, but it certainly gives some benefits, listed above.
How to get Tripadvisor Content API key
Tripadvisor Content API is granted free of charge. But getting a Tripadvisor API key for displaying their content may take some time, because the company requires correspondence to certain criteria and grants a limited number of keys.
Step 1 – Create an account
Go to the Tripadvisor service . You can sign up with your existing Facebook or Google account or create a new account.
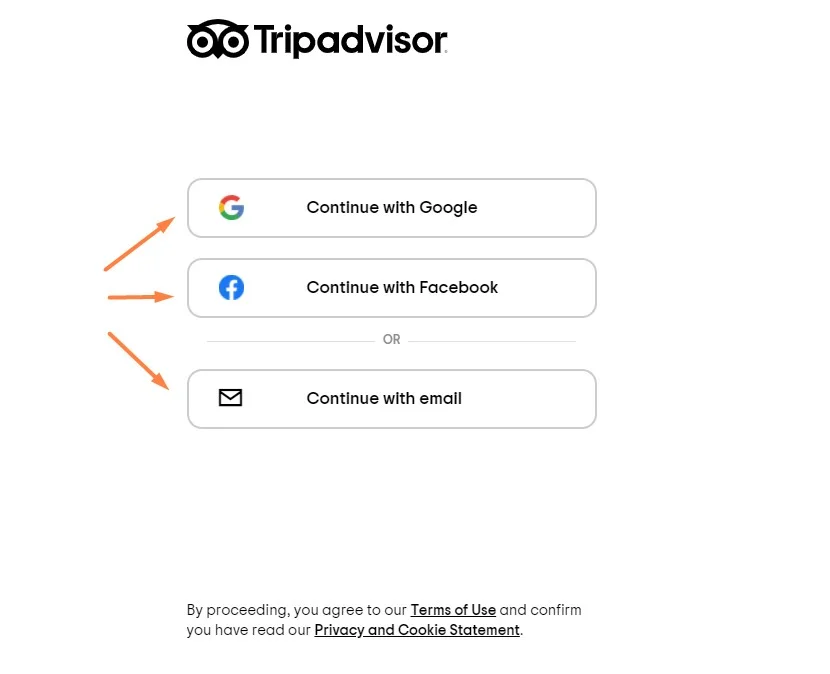
Step 2 – Pick your business needs for API key, click OK
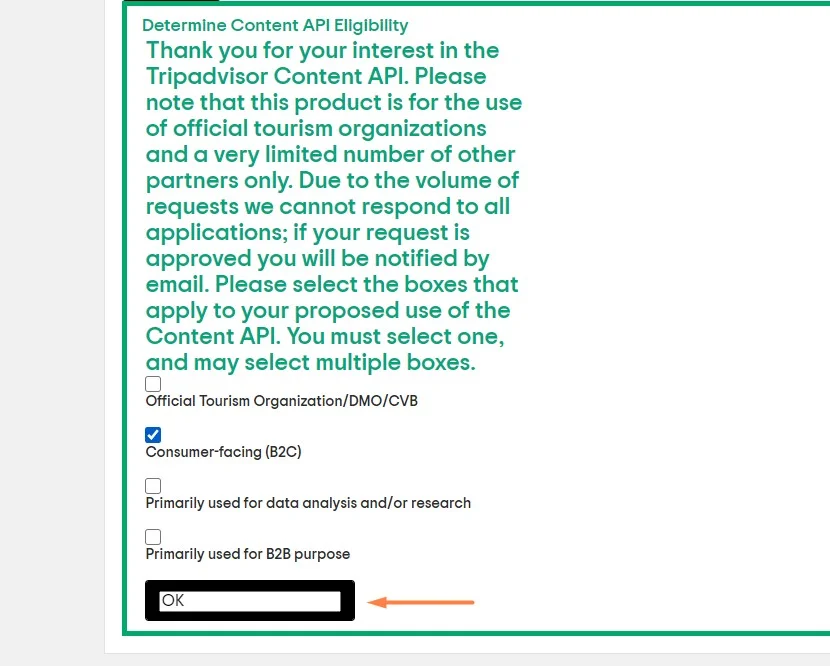
Step 3 – Then fill in the following application form
Fill each field in the form carefully.
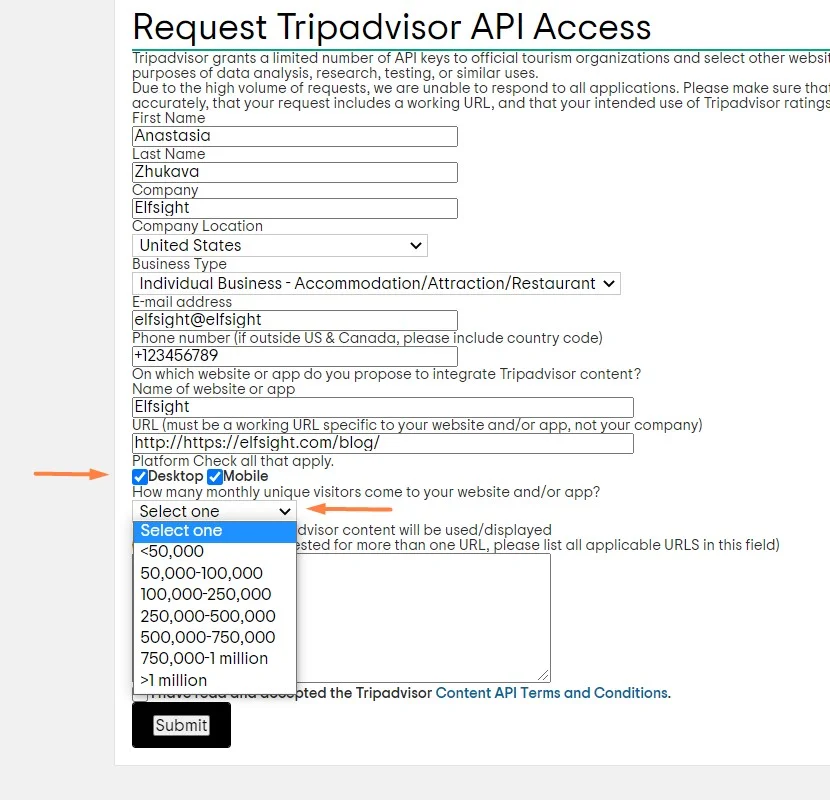
Step 4 – Describe your business plans
Don’t miss the field with the description of your use case. Tell about your plans and aims of using the content from Tripadvisor. It will increase your chances of getting approved for API Tripadvisor. Read Terms and Conditions and press Submit.
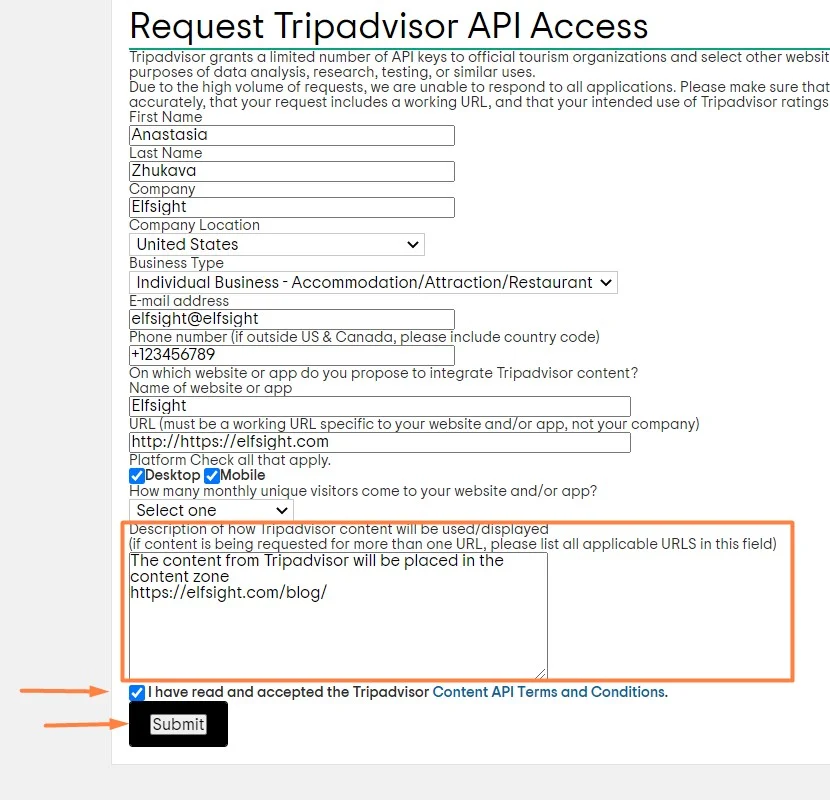
After the form submission you only have to wait to hear from Tripadvisor specialists to know whether they’ve approved your application or not.
How to get Tripadvisor TripConnect API key
If you are a pretty large tourist-oriented web business or if you represent an actual hotel or any hospitality facility, you might want to become a certified partner of Tripadvisor. The platform offers the partnership based on a commission model, which means no upfront investment is needed.
To apply for Tripadvisor TripConnect API fill and submit the following form with detailed information about your business.
Tripadvisor API examples
This kind of data cannot be accessed through the Content API implementation. But the platform provides a B2B Express Reviews API solution for easy reviews collection and processing. Tripadvisor Review API creates automated requests on behalf of a booking service asking them to leave a review and describe their experience
Tripadvisor gives an opportunity to display places’ ratings according to its data only by the means of Content API integration. If you would like to get this kind of API for your project, check the instruction above.
The affiliate program doesn’t offer any Tripadvisor affiliate API to its partners, only affiliate links. But you are free to become a partner of the service, if you are running a B2C or B2B web service. You can request a suitable API Tripadvisor key from the described above: Content API partner key for a user-facing website, or TripConnect to get a Tripadvisor affiliate hotel API, for example. This package requires no upfront payments and is based on a commission model .
Integration on a website examples
What Tripadvisor content to use and best way to integrate it on your website depends on your business type and needs. If you run a landing page for any hospitality facility, like a hotel or a restaurant, you can upgrade your website tremendously by displaying your Tripadvisor hotel reviews, API or a widget are needed. In case you run a tourist-oriented web-service or app, like an online booking engine or hotels and restaurants aggregator service, you might benefit from the Tripadvisor data like hotels availability, mapping, and prices.
How to integrate Tripadvisor API on a website, such as WordPress
The content from the platform can be integrated on any cms or site builder. There are several options for that: integration of the key into the website’s code, using Tripadvisor widget, or third-party solutions. In this article we are going to describe one of the most popular content integration cases – integration into wordpress-based websites.
Third-party solutions
Tripadvisor api website integration.
First you have to become an approved platform partner. Only after that you will get a specific kind of key, depending on the application submitted, for example, a Reviews API. When you have the key, create a custom page template and integrate the API Tripadvisor into your page template. Then you will be able to create new pages using your new page template.
TripAdvisor Widget integration
The company also offers a range of ready-made widgets for the business owners to display Tripadvisor account data right on their landing pages. Though these widgets are offered at no cost, using them isn’t usually a smooth experience. You have to implement 3 separate widgets, if you want to place Tripadvisor reviews, rating, and the button Write a review. Secondly, these widgets don’t provide any customization options at all, every user gets one and the same looking widget. Also, you have no option for reviews moderation. No need to tell this will hardly fit your website’s individual requirements and style.
Tripadvisor API pricing and limits
The Content Tripadvisor API cost is free and provided in exchange for traffic from their integrations to Tripadvisor. There are also some content display requirements you have to meet, like showing Tripadvisor logos and linking back to their website on every page. You can read the full range of requirements here .
For this type of API there’s a limit of calls available.
Latest Tripadvisor API Changes
Tripadvisor updates its API once in several years and promises upcoming changes to be released soon. The latest updates took place in 2016 with the API 2.0 version. In this version syntax and localizations were updated for a consistent names formation and standardization according to requests.
Tripadvisor API Documentation
There’s a Tripadvisor portal for developers with the description of the kinds of API provided by the Tripadvisor service. To navigate through it, choose the type you are interested in: Content API – for a customer-oriented web business, TripConnect – solutions for connectivity developed specially for B2B sphere and partners, working in hospitality industry, or APIs for self-implemented partners – those tourist facilities, that would like to be presented at and get reservations through Tripadvisor service.
My API key is not working
Make sure the key you are using is exactly the same one as the key that was provided to you via email after you’ve completed the application process.
Can I get Tripadvisor Location IDs for hotels, restaurants and other attractions?
There is a /location_mapper call available for Content API users. This call is made with a mapper-specific API key – simply your API Tripadvisor key with “-mapper” added at the end. The mapper key has a limit of 25,000 API calls daily, which allows to automate some mapping process with a script.
Can I get the Tripadvisor API key for reputation management service or B2B product?
The Content API is intended only for consumer-facing (B2C) websites and apps. For your B2B needs apply for a TripConnect partnership.
May I apply for Tripadvisor API for academic research?
Despite its zero cost, Content API cannot be used in non commercial purposes and is granted only for traffic acquiring purposes.
It might be a little difficult to understand the existing kinds of Tripadvisor API keys and even more difficult – to get one. The fact that they are provided only to approved partners, really makes Tripadvisor API access something elusive. Even affiliate the program is run without this seemingly integral part of it. But this by no means should discourage you from the intentions to display your rating and reviews Tripadvisor has collected.
Today it is possible to embed the data right from your Tripadvisor account to your own business website with Elfsight widget for Tripadvisor . You won’t have to wait for API approval and study developers documentation. It’s fast, easy, and coding-free – give it a try!
Related Posts
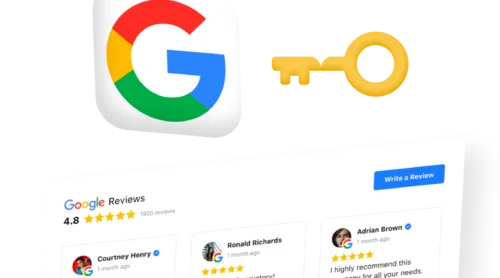
- API Description
The content below is outdated. Please follow this link to get access to our Content API.
==== outdated content ==== How can I get an API key?
First review our terms of use and content display guidelines to determine if you are qualified to apply for a Content API key. If you meet the criteria, you may submit an application online: https://www.tripadvisor.com/APIAccessSupport
What do I do if my API key is not working?
Verify that the key you are attempting to use is exactly the same as the key you were provided via email when you completed the sign-up process.
What do I do if my API key has expired?
If you received a provisional key more than six (6) months ago and have not yet contacted the Content API Team with a proposed integration, or received permission from Tripadvisor to launch your integration, you have exceeded the maximum development time and your key will be terminated.
If you are still interested in utilizing the Content API and meet the guidelines set forth in the Developer Portal, please resubmit your application. Please remember that you must:
- Have a working URL for your consumer-facing, travel-related website/app
- Plan to develop and launch your integration of Tripadvisor content within six (6) months of receiving a provisional key
Can I request a higher rate limit?
Tripadvisor sets a limit of 50 calls/second and 1,000 calls per-day to developers using our Content API during the period of development and QA. Once the application is approved for launch, Tripadvisor will increase the daily limit to 10,000 calls.
How can I get Tripadvisor Location IDs for hotels, restaurants and attractions?
Tripadvisor does not assist with the provision of property Location IDs, however there is a /location_mapper call for Content API users. This call can be made using a mapper-specific API key, which is just your API key plus "-mapper". So, if your key was "abcdef123", your mapper-specific key would be "abcdef123-mapper". This mapper key has a per day limit of 25,000 API calls and a per second limit of 100 API calls, allowing you to automate some of your mapping process with a well-written script. More information can be found here .
All Content API user mapping options can be found here .
Does Tripadvisor have any code libraries for the Content API?
Tripadvisor does not have code libraries for its Content API.
Can I access the API in order to add Tripadvisor data in my reputation management tool or B2B product?
No - the Tripadvisor Content API is intended for consumer-facing (B2C) websites & apps only.
My site does not have a working URL - can I apply?
Websites and apps without working URLs are not eligible for a Content API key. Please resubmit your application once your site has gone live and established a predictable monthly visitor base.
Can I access the API for my academic research?
Do I need to send Tripadvisor a test site/app with my proposed integration and receive permission to display content on my live website if I don't need more than 1,000 calls per day?
Yes - all proposed integrations must be sent to Tripadvisor for approval to ensure that all display requirements are met. If you do not submit a request to go live within six (6) months of receiving your provisional key, your API access will be terminated.
How do I get more than 1,000 calls for use in development?
Your provisional API key is to be used for development purposes only and is limited to 1,000 calls per day. Call volume will not be increased until the integration of Tripadvisor content on your test-site is approved.
Does the Content API include vacation rental content?
No - vacation rental content, including ratings and reviews, are not available via the Content API.

IMAGES
COMMENTS
The Tripadvisor Review Express API enables connectivity partners to provide automated Review Express services to opted-in hotel partners — creating requests on their behalf to send emails to recent guests, encouraging a review of their experiences. To learn more about Review Express and how your hotel partners can benefit, click here.
The Partner API provides provide dynamic access to Tripadvisor content. Partners can integrate the content into their websites and applications by calling the API, parsing the response, and displaying the data from the response on their site or in their app. Approved users of the Tripadvisor Content API can access the following business details ...
Checklist for Implementing Review Express API. To test and enable the Review Express API, please follow the steps outlined in the checklist below: ☐ Complete your TripConnect™ or Review Express connectivity hotel mapping. TripConnect Partners: To get started, your business must be certified as a TripConnect Partner with a valid, completed ...
Three views for the web app. Connect to the Tripadvisor API. Step 2. Set up Node, Express, and Handlebars. To make the three static pages above, we're going to have to install Node.js, Express.js, and Handlebars.js.In the table above, there is a link to instructions and information for the set of each, but in a nutshell, you will need to open your terminal, create a folder and use the ...
The Tripadvisor Content API gives developers access to Tripadvisor's highly recognized and trusted data set of more than 7.5 million locations, 1 billion reviews & opinions, and 29 languages. How can I get started using the Tripadvisor Content API?
We would like to show you a description here but the site won't allow us.
Our partner API provides you with dynamic access to Tripadvisor content, enabling seamless integration with your website and applications. Locations are defined within this API as hotels, restaurants or attractions. Get access to location details and up to 5 reviews and 5 photos per location; Up to 50 calls per second; Pay only for what you use
In general, travelers want to share great experiences — 82% of reviews on Tripadvisor receive 4 or 5 bubbles. Snippets provide a quick snapshot with a word or phrase that adds context to the initial bubble rating. These might be phrases like, "Great place for a date night!" or "Our kids loved the pool!". Long-form reviews offer ...
Hello, I have some questions regarding the retrieval of reviews via API. (1) Can I retrieve more than 5 reviews using the API? If yes, How? (2) is the trip_type (e.g. Business) the only filter that I can use?
Demystifying the TripAdvisor Content API: Your Gateway to Seamless Data Integration The TripAdvisor Content API, formerly the TripAdvisor API, offers an official avenue for scraping TripAdvisor data. A significant evolution from previous years, the TripAdvisor Content API streamlines the process, requiring less rigorous vetting for access.
How to implement trip advisor api to get rating and review about a specific hotel. 1. Scrapy, Crawling Reviews on Tripadvisor: extract more hotel and user information. 8. How to get all google reviews using business api. 36. API to get all the reviews and rating from Google for business. 0.
How to implement trip advisor api to get rating and review about a specific hotel. 1 Scrapy, Crawling Reviews on Tripadvisor: extract more hotel and user information. 2 Review scraping form tripadvisor. 36 API to get all the reviews and rating from Google for business ...
Increase your user experience, engagement, and conversion with Tripadvisor's globally recognized and highly trusted content. Our partner API provides you with dynamic access to Tripadvisor content, enabling seamless integration with your website and applications. Get access to business details and up to 3 reviews and 2 photos per location
Using an API (Application Programming Interface) or an HTML module, you can get the required quickly and smoothly. and quickly. Read this blog and learn about why these TripAdvisor reviews are so important, what web scraping is, as well as how you can extract reviews data from TripAdvisor using an HTML module or an API.
Hello, I have some questions regarding the retrieval of reviews via API. (1) Can I retrieve more than 5 reviews using the API? If yes, How? (2) is the trip_type (e.g. Business) the only filter that I can use?
Scraping Reviews From TripAdvisor With an API. An API will allow you to scrape through web pages in real-time, instead of individually entering each page you want to scrape. An API is a software interface that connects different computer programs and allows them to transfer data without exposing the code underlying each data transfer. By ...
Tripadvisor reviews API. This kind of data cannot be accessed through the Content API implementation. But the platform provides a B2B Express Reviews API solution for easy reviews collection and processing. Tripadvisor Review API creates automated requests on behalf of a booking service asking them to leave a review and describe their experience.
How to implement trip advisor api to get rating and review about a specific hotel. 8. How to get all google reviews using business api. 36. API to get all the reviews and rating from Google for business. 5. How to get more than 3 reviews from Yelp API request. Hot Network Questions
Reviews API - Tripadvisor Support Forum. Tripadvisor Forums ; Tripadvisor Support Forums; Search. Browse all 70,997 Tripadvisor Support topics » ... TripAdvisor now makes free rating, review, and listing content available to qualified consumer-facing (B2C) websites though its Content API.
Approved users of the Tripadvisor Content API can access the following business details for accommodations, restaurants, and attractions: Location ID, name, address, latitude & longitude. Read reviews link, write-a-review link. Overall rating, ranking, subratings, awards, the number of reviews the rating is based on, rating bubbles image.
Tripadvisor's approach to reviews. Before posting, each Tripadvisor review goes through an automated tracking system, which collects information, answering the following questions: how, what, where and when. If the system detects something that potentially contradicts our community guidelines, the review is not published.
Book Maison Boissiere By Barnes, Paris on Tripadvisor: See traveller reviews, 7 candid photos, and great deals for Maison Boissiere By Barnes, ranked #1,546 of 1,846 hotels in Paris and rated 5 of 5 at Tripadvisor.
Tripadvisor sets a limit of 50 calls/second and 1,000 calls per-day to developers using our Content API during the period of development and QA. Once the application is approved for launch, Tripadvisor will increase the daily limit to 10,000 calls.
How to get all reviews from Trip advisor api. 0. Can users rate a restaurant by using google places API? 36. API to get all the reviews and rating from Google for business. 2. Get property rating from Booking.com API. Hot Network Questions Common Indoeuropean Phonotactics Rules
Detailed Reviews: Reviews ordered by recency and descriptiveness of user-identified themes such as waiting time, length of visit, general tips, and location information. We perform checks on reviews. Tripadvisor's approach to reviews.
Does anyone know a good way of contacting the trip advisor API team. I keep sending emails... but I feel like I'm going in circles and getting nowhere fast. ... Tripadvisor reviews + API. 1 reply. Request for TripAdvisor API. 3 replies. TripAdvisor API: Retrieve reviews. 3 replies. TripAdvisor API not returning the latest data for CN. 1 reply ...
Most Recent: Reviews ordered by most recent publish date in descending order. Detailed Reviews: Reviews ordered by recency and descriptiveness of user-identified themes such as wait time, length of visit, general tips, and location information. Excellent. 0. Very good. 1. Average. 0. Poor. 0. Terrible. 0.